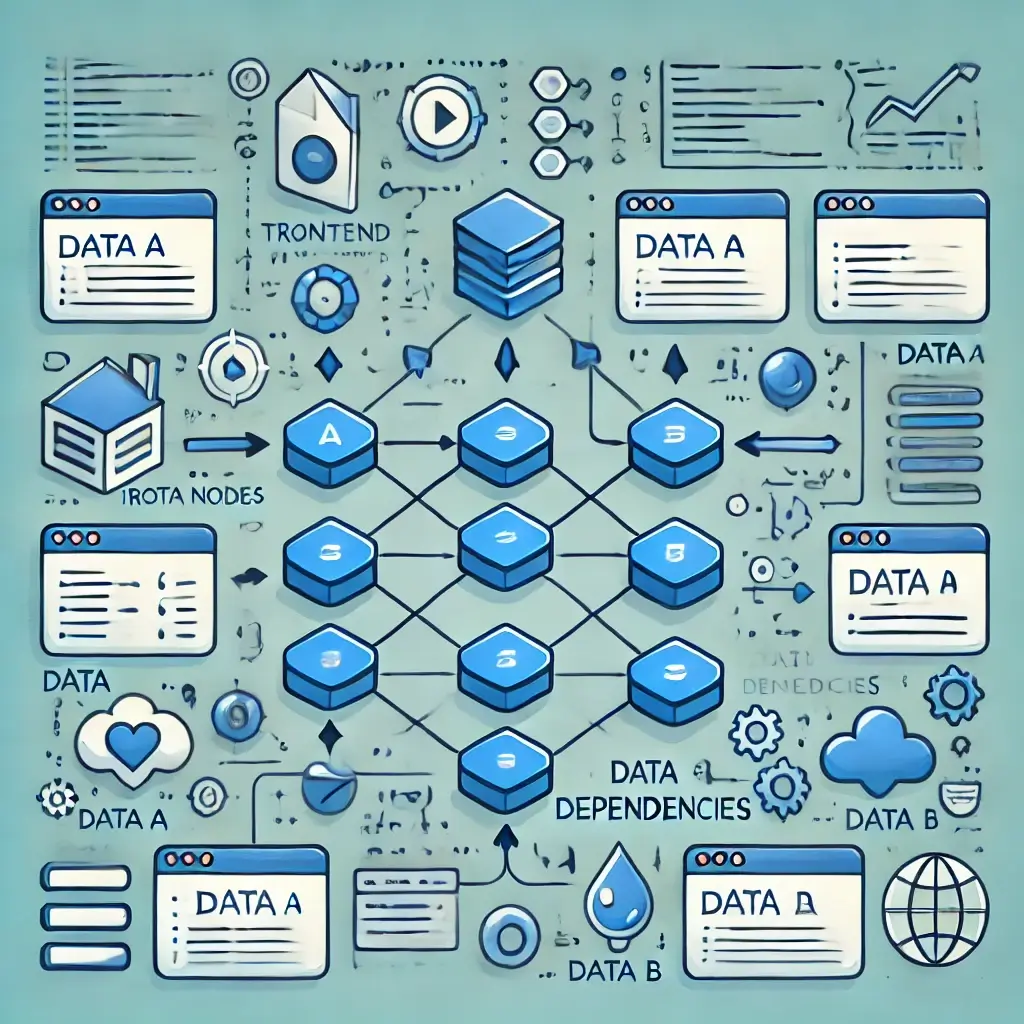
Understanding Transitive Data Dependencies in Frontend Applications
In frontend development, data dependencies refer to the relationships between various data sources or states within an application. A transitive data dependency occurs when a piece of data indirectly depends on another piece of data. For instance, if a component relies on data A, which itself depends on data B, then the component has a transitive dependency on data B.
These dependencies can arise in various scenarios, such as when fetching data from APIs, syncing local and remote state, or using state management libraries like Redux, MobX, or Vuex. Understanding and managing these transitive data dependencies is crucial for ensuring data consistency and optimal performance in frontend applications.
How Frontend Applications Know When to Refetch Transitive Data Dependencies
Frontend applications use several mechanisms to determine when transitive data dependencies need to be refetched:
-
Reactive Programming and Observables: Many modern frontend frameworks (such as React with hooks, Vue with its reactivity system, or Angular with RxJS) use reactive programming principles. In these systems, data dependencies are often tracked automatically. When a change occurs in a source data (e.g., data B), the framework automatically triggers updates for any dependent data (e.g., data A) and components that rely on it. This automatic propagation of changes allows the application to “know” when to refetch or recompute dependent data.
-
State Management Libraries: Libraries like Redux, MobX, and Vuex help manage application state more predictably. They often use a central store to maintain the state, and changes to the state are tracked through actions or mutations. When a piece of state changes, these libraries can automatically update all dependent states and components. If a transitive dependency changes (e.g., data B), the library can trigger a recomputation or refetch of any state that indirectly depends on it (e.g., data A).
-
Cache Invalidation Strategies: In applications that rely heavily on remote data fetching (such as REST APIs or GraphQL), cache invalidation strategies are used to determine when data should be refetched. Tools like React Query, Apollo Client, or SWR provide mechanisms to cache data locally and invalidate the cache when certain conditions are met. For instance, if data B is updated and data A is a derived state from data B, the cache invalidation strategy might dictate that data A needs to be refetched or recomputed based on the updated data B.
-
WebSocket and Real-Time Updates: In applications requiring real-time data synchronization, such as chat apps or live dashboards, WebSocket connections or similar real-time communication protocols are used. When the backend sends an update to the client (e.g., data B changes), the frontend can automatically trigger updates for any transitive dependencies. This approach ensures that all dependent data remains in sync without the need for manual polling or checks.
How Are Transitive Data Dependencies Updated and Kept in Sync in Memory?
Keeping transitive data dependencies updated and in sync in memory involves several strategies:
-
Reactive State Management: As mentioned earlier, using reactive state management systems (like React’s useState and useEffect hooks, Vue’s reactive system, or Angular’s RxJS observables) allows applications to automatically update dependent data. When a state changes, all components or states that depend on it are re-evaluated or re-rendered. This automatic reactivity ensures that all transitive dependencies are kept up to date in memory.
-
Selectors and Derived State: In state management libraries, selectors are often used to compute derived states. For example, if data A depends on data B, and data B changes, a selector can recompute data A based on the new value of data B. This recomputation happens in memory, ensuring that all dependent states are updated without redundant re-fetches from external sources.
-
Memoization Techniques: Memoization is a technique used to optimize expensive computations by caching the results of function calls. In the context of transitive data dependencies, memoization can prevent unnecessary recomputations of derived data when only non-relevant dependencies change. For example, React’s
useMemo
hook or libraries like Reselect in Redux can memoize computed data, ensuring efficient updates in memory. -
Real-Time Synchronization: For applications relying on real-time data, synchronization mechanisms like WebSockets or server-sent events ensure that any change in the underlying data is instantly propagated to all dependent states. This approach keeps all data dependencies in sync in memory without delay, providing a seamless real-time user experience.
-
Optimistic Updates and Rollbacks: When updating data, some frontend applications use optimistic updates to immediately reflect changes in the UI while the actual update request is sent to the server. If the update fails, the application can roll back to the previous state. This approach helps maintain a responsive user experience and ensures that transitive dependencies are updated in memory promptly, reducing perceived latency.
-
Client-Side Caching and Stale-While-Revalidate: Tools like React Query or SWR provide advanced caching strategies, such as stale-while-revalidate, which allows data to be served from the cache while a background fetch updates it. When data changes (e.g., data B), these tools refetch and update all dependent data (e.g., data A) in memory, ensuring data consistency without blocking the UI.
Conclusion
Managing transitive data dependencies in frontend applications is essential for maintaining data consistency, performance, and a smooth user experience. By leveraging reactive programming principles, state management libraries, real-time synchronization, and advanced caching strategies, developers can ensure that all data dependencies are kept up-to-date and in sync in memory. Understanding these concepts helps build robust applications that handle data efficiently, reduce unnecessary re-fetches, and provide users with a responsive and reliable experience.