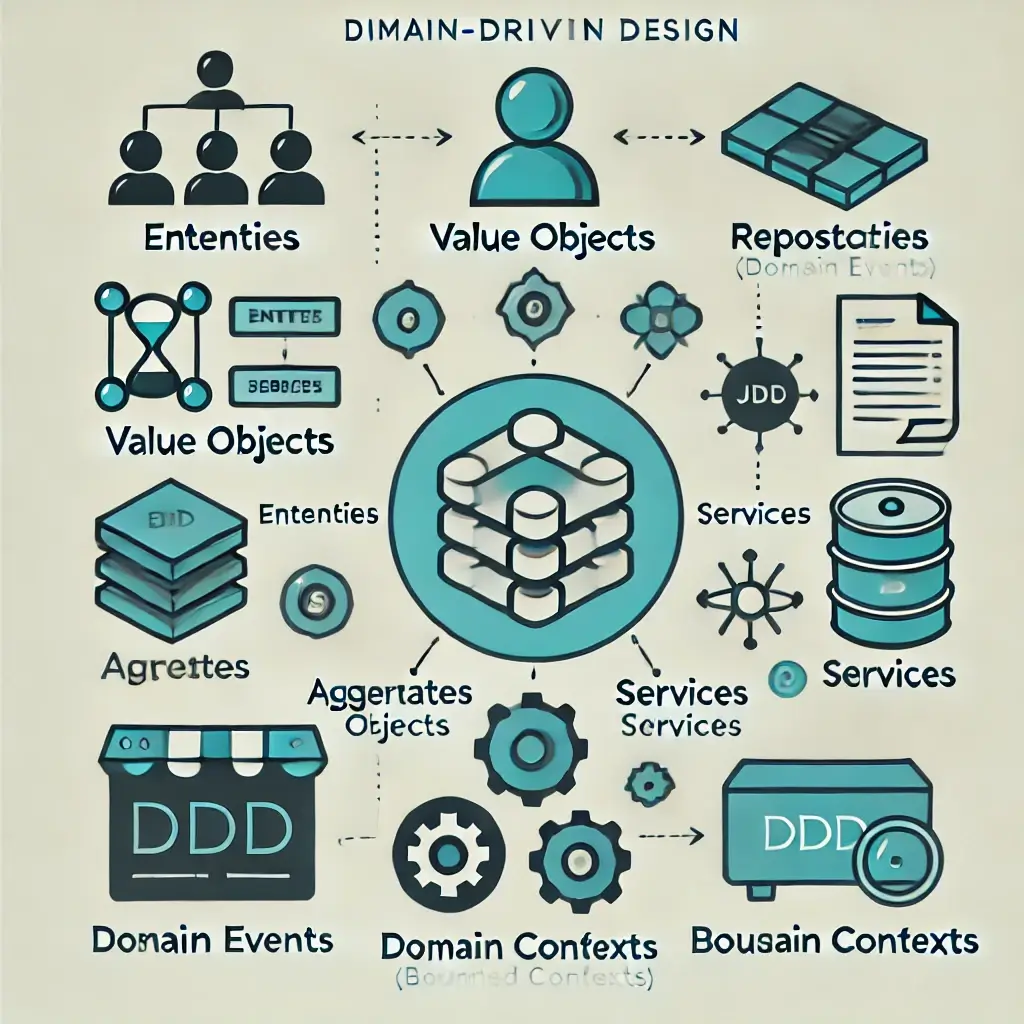
Understanding Domain-Driven Design (DDD) and How to Leverage It in Software Development
Domain-Driven Design (DDD) is a software design methodology that focuses on modeling software around the core business domain. Introduced by Eric Evans in his seminal book Domain-Driven Design: Tackling Complexity in the Heart of Software, DDD emphasizes creating a shared understanding between domain experts and developers to ensure the software reflects the complexities of the business domain.
DDD is particularly useful in complex systems where understanding and managing the domain is crucial. By aligning the software design with business rules, processes, and knowledge, DDD helps create software that is more maintainable, scalable, and adaptable to change.
Core Concepts of Domain-Driven Design
To effectively apply Domain-Driven Design, it’s crucial to understand its core concepts:
-
Domain: The domain represents the core area of knowledge or activity that the software is designed to address. It encompasses all business logic, rules, and processes specific to that context.
-
Entities and Value Objects:
- Entities are objects with a distinct identity and lifecycle. They represent concepts that can change over time but remain uniquely identifiable, such as a
Customer
orOrder
. - Value Objects are objects defined by their attributes rather than identity. They are immutable and often represent concepts like
Money
,DateRange
, orAddress
.
- Entities are objects with a distinct identity and lifecycle. They represent concepts that can change over time but remain uniquely identifiable, such as a
-
Aggregates and Aggregate Roots: An aggregate is a group of related entities and value objects treated as a single unit for data changes. The aggregate root is the main entity that controls access to the aggregate and ensures data integrity within its boundaries.
-
Repositories: Repositories are abstractions over data storage, responsible for retrieving and persisting aggregates. They allow developers to focus on domain logic instead of data persistence concerns.
-
Services: Domain services contain operations that do not naturally fit within an entity or value object. They represent domain logic that applies across multiple aggregates or involves operations not tied to a specific entity.
-
Domain Events: Domain events are notifications that something significant has happened within the domain, such as “Order Placed” or “Payment Processed.” They help decouple parts of the system by allowing different components to react to changes in the domain.
-
Bounded Contexts: A bounded context is a defined boundary within which a particular domain model is valid. It helps manage complexity by dividing the domain into smaller, more manageable parts, each with its own model and language.
Why Use Domain-Driven Design in Software Development?
Domain-Driven Design offers several benefits across various types of software development projects:
-
Improved Alignment with Business Goals: By focusing on the domain, DDD ensures that the software accurately reflects real business needs and processes, reducing the gap between developers and domain experts.
-
Enhanced Code Organization and Maintainability: DDD encourages a clear separation between domain logic and technical concerns (like data persistence or UI handling), leading to more organized and maintainable code.
-
Facilitates Collaboration: DDD promotes a shared language (Ubiquitous Language) that both developers and domain experts use, improving communication and collaboration and ensuring the software meets business requirements.
-
Adaptability to Change: As business needs evolve, a domain-driven approach makes it easier to adapt the software by changing the domain model without necessarily overhauling the entire application.
Leveraging Domain-Driven Design in Software Development
To effectively leverage Domain-Driven Design in your software projects, follow these steps:
1. Collaborate with Domain Experts to Define the Domain Model
Start by working closely with domain experts to gain a deep understanding of the business domain. Identify key entities, value objects, aggregates, and services. The goal is to create a domain model that accurately reflects the business rules and processes.
Example: E-Commerce Platform
For an e-commerce platform, the domain model might include entities such as Customer
, Product
, and Order
, and value objects like Money
(to handle currency and amount) or ShippingAddress
.
# Value Object: Money
class Money:
def __init__(self, amount, currency):
self.amount = amount
self.currency = currency
def add(self, other):
if self.currency != other.currency:
raise ValueError("Currencies do not match")
return Money(self.amount + other.amount, self.currency)
def __str__(self):
return f"{self.amount} {self.currency}"
# Entity: Customer
class Customer:
def __init__(self, customer_id, name, email):
self.customer_id = customer_id
self.name = name
self.email = email
def update_email(self, new_email):
# Business rule: validate email format
self.email = new_email
def __str__(self):
return f"Customer({self.customer_id}): {self.name}, {self.email}"
2. Identify Bounded Contexts
Break down the domain into multiple bounded contexts, each representing a specific sub-domain or feature of the application. A bounded context defines a clear boundary within which a particular domain model is consistent and meaningful.
Each bounded context should have its own model and language, which helps manage complexity and prevents ambiguity.
Example: Inventory Management and Sales
In an e-commerce platform, you might have separate bounded contexts for “Inventory Management” and “Sales”. The Product
entity in “Inventory Management” might include attributes like quantityInStock
and warehouseLocation
, while the same Product
entity in the “Sales” context might have attributes like price
and discount
.
3. Design Domain Entities and Value Objects
Implement domain entities and value objects that encapsulate the core business logic. These classes should ensure that business rules are enforced consistently and should be designed to be easily testable and maintainable.
Example: Order Aggregate
For an e-commerce platform, the Order
aggregate might include multiple OrderItem
entities, each representing a product included in the order. The Order
entity would be the aggregate root, ensuring that all operations on OrderItem
entities are consistent.
# Value Object: OrderItem
class OrderItem:
def __init__(self, product, quantity, unit_price):
self.product = product
self.quantity = quantity
self.unit_price = unit_price
def total_price(self):
return self.quantity * self.unit_price
# Entity: Order (Aggregate Root)
class Order:
def __init__(self, order_id, customer):
self.order_id = order_id
self.customer = customer
self.items = []
self.status = 'Pending'
def add_item(self, product, quantity, unit_price):
# Business rule: Ensure quantity is available
item = OrderItem(product, quantity, unit_price)
self.items.append(item)
def total_amount(self):
return sum(item.total_price() for item in self.items)
def complete_order(self):
# Business rule: Can only complete if status is 'Pending'
if self.status != 'Pending':
raise ValueError("Order is not in a valid state to complete.")
self.status = 'Completed'
def __str__(self):
return f"Order({self.order_id}) for {self.customer.name}: {self.status}, Total: {self.total_amount()}"
4. Define Aggregates and Aggregate Roots
Organize your domain model into aggregates to enforce consistency boundaries. Ensure that each aggregate is managed through its aggregate root, which controls access to its internal state and maintains invariants.
Example: Inventory Aggregate
In an inventory management system, the Inventory
aggregate could be managed through an InventoryItem
aggregate root that controls operations like adding or removing stock and ensures that stock levels do not fall below zero.
# Entity: InventoryItem (Aggregate Root)
class InventoryItem:
def __init__(self, item_id, product, quantity):
self.item_id = item_id
self.product = product
self.quantity = quantity
def add_stock(self, amount):
self.quantity += amount
def remove_stock(self, amount):
if amount > self.quantity:
raise ValueError("Insufficient stock to remove.")
self.quantity -= amount
def __str__(self):
return f"InventoryItem({self.item_id}): {self.product.name}, Quantity: {self.quantity}"
5. Implement Repositories for Data Persistence
Create repositories to handle data persistence and retrieval for aggregates. Repositories provide an abstraction over the data storage mechanism, allowing you to focus on domain logic rather than the specifics of data handling.
Example: CustomerRepository
For an e-commerce platform, a CustomerRepository
might handle operations such as finding customers by ID or saving new customers to the database.
# Repository for managing customer data
class CustomerRepository:
def __init__(self, data_source):
self.data_source = data_source
def find_by_id(self, customer_id):
# Logic to retrieve customer from data source
customer_data = self.data_source.get(customer_id)
if not customer_data:
return None
return Customer(**customer_data)
def save(self, customer):
# Logic to save customer to data source
self.data_source[customer.customer_id] = {
'name': customer.name,
'email': customer.email
}
# Other repository methods...
6. Use Domain Services for Cross-Aggregate Operations
Implement domain services for operations that span multiple aggregates or that don’t naturally fit within an entity or value object. Domain services should be stateless and contain only domain logic.
Example: PaymentService
For an e-commerce platform, a PaymentService
might handle the payment process for orders, which involves multiple aggregates like Order
and Customer
.
# Domain service for handling payment processing
class PaymentService:
def __init__(self, payment_gateway, order_repository, customer_repository):
self.payment_gateway = payment_gateway
self.order_repository = order_repository
self.customer_repository = customer_repository
def process_payment(self, order_id, payment_details):
order = self.order_repository.find_by_id(order_id)
if not order or order.status != 'Pending':
raise ValueError("Order cannot be processed.")
customer = self.customer_repository.find_by_id(order.customer.customer_id)
if not customer:
raise ValueError("Customer not found.")
# Business rule: Validate payment details, calculate total, and charge
if self.payment_gateway.charge(payment_details, order.total_amount()):
order.complete_order()
self.order_repository.save(order)
return True
else:
return False
7. Leverage Domain Events for Decoupling and Asynchronous Processing
Domain events are a powerful tool for decoupling different parts of your application and supporting asynchronous processing. When significant changes occur within your domain, emit domain events that other parts of your system can react to.
Example: OrderPlacedEvent
When an order is placed, an OrderPlacedEvent
can be emitted to notify other parts of the system, such as inventory management or customer notification services.
# Domain event class
class DomainEvent:
def __init__(self, name, data):
self.name = name
self.data = data
# Example of emitting a domain event when an order is placed
class Order:
# Previous methods...
def place_order(self):
# Business logic for placing an order
self.status = 'Placed'
order_placed_event = DomainEvent("OrderPlaced", {"order_id": self.order_id})
event_dispatcher.dispatch(order_placed_event)
8. Continually Refactor and Evolve the Domain Model
Domain-Driven Design is an iterative process. As you learn more about the domain and as business requirements change, continually refactor and evolve your domain model to ensure it accurately reflects the current understanding of the domain.
Conclusion
Domain-Driven Design offers a robust methodology for managing complexity in software development by focusing on the core business domain. By defining a clear domain model, identifying bounded contexts, organizing domain entities and value objects, leveraging repositories and services, and utilizing domain events, you can build software that is more aligned with business needs, easier to maintain, and more adaptable to change.
Whether you are developing a front-end, back-end, or full-stack application, applying DDD principles helps create a more structured, organized, and effective codebase that grows and evolves with your business.