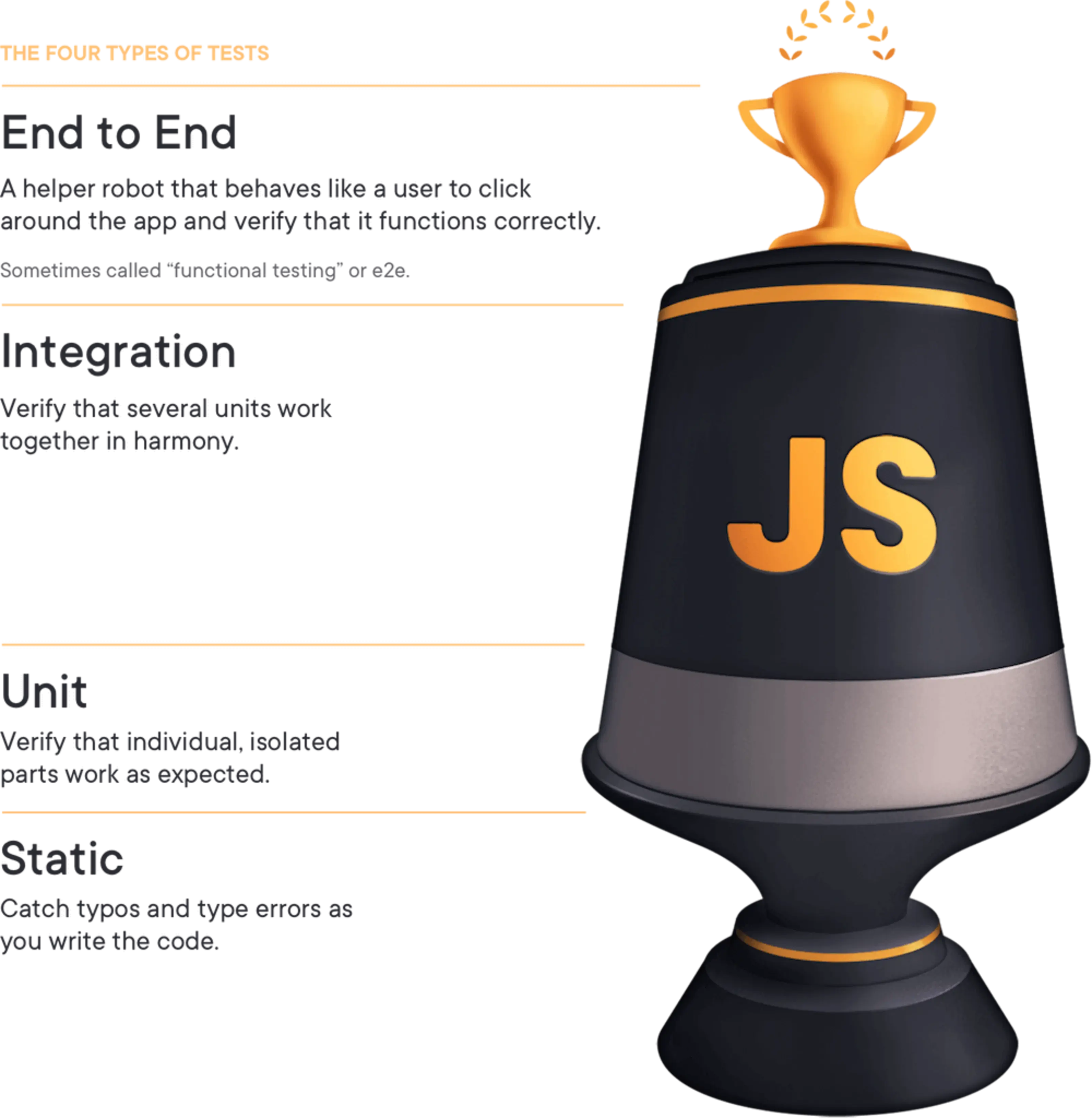
Static vs. Unit vs. Integration vs. E2E Testing for Frontend Apps
In software development, testing is crucial for ensuring that applications are reliable, secure, and perform well. By incorporating a comprehensive testing strategy, developers can detect errors early, save time and money, and increase customer satisfaction. When it comes to frontend applications, choosing the right types of tests is essential for delivering business value efficiently. This is where the Testing Trophy, created by Kent C. Dodds, provides a clear framework to guide developers.
The Testing Trophy outlines four key types of tests, ranked in a hierarchy from bottom to top, reflecting the focus each should receive in a well-rounded testing strategy:
- Static: Catches typos and type errors as you write code.
- Unit: Verifies that individual, isolated parts of the code work as expected.
- Integration: Ensures that several units work together harmoniously.
- End-to-End (E2E): Simulates user interactions to verify that the entire app functions correctly.
By understanding and applying these testing types, developers can maximize the return on investment in testing while ensuring that their applications meet business goals.
Test Types Explained
End-to-End (E2E) Testing
E2E tests are vital for ensuring that the application behaves as expected from a user’s perspective. They validate complete workflows and user journeys, covering both frontend and backend systems. This holistic approach gives the highest confidence that the application will function correctly in a production environment.
E2E tests simulate real-world scenarios, such as user registration, login, or completing a transaction, ensuring that all parts of the system integrate well together. Here’s an example using Cypress:
import { generate } from 'todo-test-utils';
describe('todo app', () => {
it('login and complete a todo', () => {
// here we generate needed data to interact with the frontend, a new user and a new todo
const user = generate.user();
const todo = generate.todo();
// open the application
cy.visitApp();
// login with the user's credentials
cy.findByText(/register/i).click();
cy.findByLabelText(/username/i).type(user.username);
cy.findByLabelText(/password/i).type(user.password);
cy.findByText(/login/i).click();
// add a new todo using the data generated earlier
cy.findByLabelText(/add todo/i).type(todo.description).type('{enter}');
// check for description being there
cy.findByTestId('todo-0').should('have.value', todo.description);
// check for todo to be completed when clicking complete
cy.findByLabelText('complete').click();
cy.findByTestId('todo-0').should('have.class', 'complete');
});
});
Trade-offs: E2E tests offer the highest confidence but are typically slower and more costly to maintain due to their broad coverage and complexity. This makes them ideal for critical business paths that require high reliability but impractical for exhaustive coverage of all edge cases.
Integration Testing
Integration tests ensure that different components or units within your application work together as intended. These tests are particularly valuable for validating critical interactions between components, such as data flow between the UI and backend services.
Integration tests aim to minimize mocking, thus providing a more realistic assessment of how components function together. Here’s an example using React Testing Library:
import * as React from 'react';
import { render, screen, waitForElementToBeRemoved } from 'test/app-test-utils';
import userEvent from '@testing-library/user-event';
import App from '../app';
test(`logging in displays the user's username`, async () => {
await render(<App />, { route: '/login' });
const username = 'testUser';
const password = 'password123';
userEvent.type(screen.getByLabelText(/username/i), username);
userEvent.type(screen.getByLabelText(/password/i), password);
userEvent.click(screen.getByRole('button', { name: /submit/i }));
await waitForElementToBeRemoved(() => screen.getByLabelText(/loading/i));
expect(screen.getByText(username)).toBeInTheDocument();
});
Trade-offs: Integration tests strike a good balance between confidence and maintainability. While they don’t provide the full coverage of an E2E test, they are faster and more focused, making them an efficient choice for validating key interactions in the application. They are also much faster since they run against virtual doms and not an entire browser engine.
Unit Testing
Unit tests focus on the smallest parts of your application, such as individual functions or components, in isolation. These tests are invaluable for catching bugs early in the development process, ensuring that individual pieces of logic perform as expected.
Unit tests are quick to run and inexpensive to write and maintain. They provide immediate feedback during development, allowing developers to iterate rapidly. Here’s an example of a unit test:
import '@testing-library/jest-dom/extend-expect';
import * as React from 'react';
import { render, screen } from '@testing-library/react';
import ItemList from '../item-list';
test('renders "no items" when the item list is empty', () => {
render(<ItemList items={[]} />);
expect(screen.getByText(/no items/i)).toBeInTheDocument();
});
test('renders the items in a list', () => {
render(<ItemList items={['apple', 'orange', 'pear']} />);
expect(screen.getByText(/apple/i)).toBeInTheDocument();
expect(screen.getByText(/orange/i)).toBeInTheDocument();
expect(screen.getByText(/pear/i)).toBeInTheDocument();
});
Trade-offs: While unit tests provide the fastest feedback and are the cheapest to maintain, they offer the lowest level of confidence since they test components in isolation. They are ideal for catching low-level bugs but should be supplemented with integration and E2E tests for comprehensive coverage.
Static Testing
Static tests involve using tools like linters and type checkers to catch errors in your code without running the application. These tools provide immediate feedback on potential issues, such as syntax errors or type mismatches, as you write your code.
Static testing is crucial for maintaining code quality and preventing simple errors from reaching production. For example, using ESLint can catch issues like this:
for (var i = 0; i < 10; i--) {
console.log(i);
}
Trade-offs: Static analysis tools are fast and provide immediate feedback, making them essential for catching errors early in the development process. However, they cannot verify the functionality or logic of the application, so they should be used in conjunction with other types of testing.
Why Do We Test?
The primary reason for writing tests is to build confidence. Confidence that the changes you make today won’t break your application in production. Effective testing enables continuous delivery of high-quality software that meets business requirements, ultimately leading to greater customer satisfaction and trust.
Trade-offs in Testing
There are three main trade-offs when it comes to testing:
- Cost: Moving up the Testing Trophy (from static to E2E), the cost of writing and maintaining tests increases.
- Speed: Tests higher up in the Trophy take longer to run because they cover more of the application.
- Confidence: Higher-level tests provide more confidence that your application works correctly but are slower and more expensive.
Finding the right balance between these factors is key to an effective testing strategy. A mix of different types of tests provides a comprehensive approach that maximizes coverage while minimizing costs and time.
Conclusion
Each level of testing offers unique benefits and trade-offs. End-to-end tests provide the highest confidence but are slower and more expensive, making them suitable for critical business paths. Integration tests offer a good balance of confidence and speed for verifying component interactions. Unit tests are fast and cheap but provide lower confidence, focusing on individual parts of the application. Static tests are essential for early detection of errors and maintaining code quality.
By understanding these trade-offs and strategically applying different testing types, you can ensure that your frontend applications are robust, maintainable, and deliver great user experiences. The goal is not to adhere strictly to one type of test but to achieve a level of confidence that meets your business needs.
For more insights on testing strategies, you can read Kent C. Dodds’ full blog post on Static vs Unit vs Integration vs E2E Tests.
Happy testing!
Further resources
- Unit, Integration, and End-to-End Testing: What’s the Difference? | Twilio
- Testing - Microsoft Engineering Fundamentals Playbook
- Unit vs Integration vs System vs E2E Testing - Engineering Fundamentals Playbook
- Unit Testing - Engineering Fundamentals Playbook
- Integration Testing - Engineering Fundamentals Playbook
- E2E Testing - Engineering Fundamentals Playbook