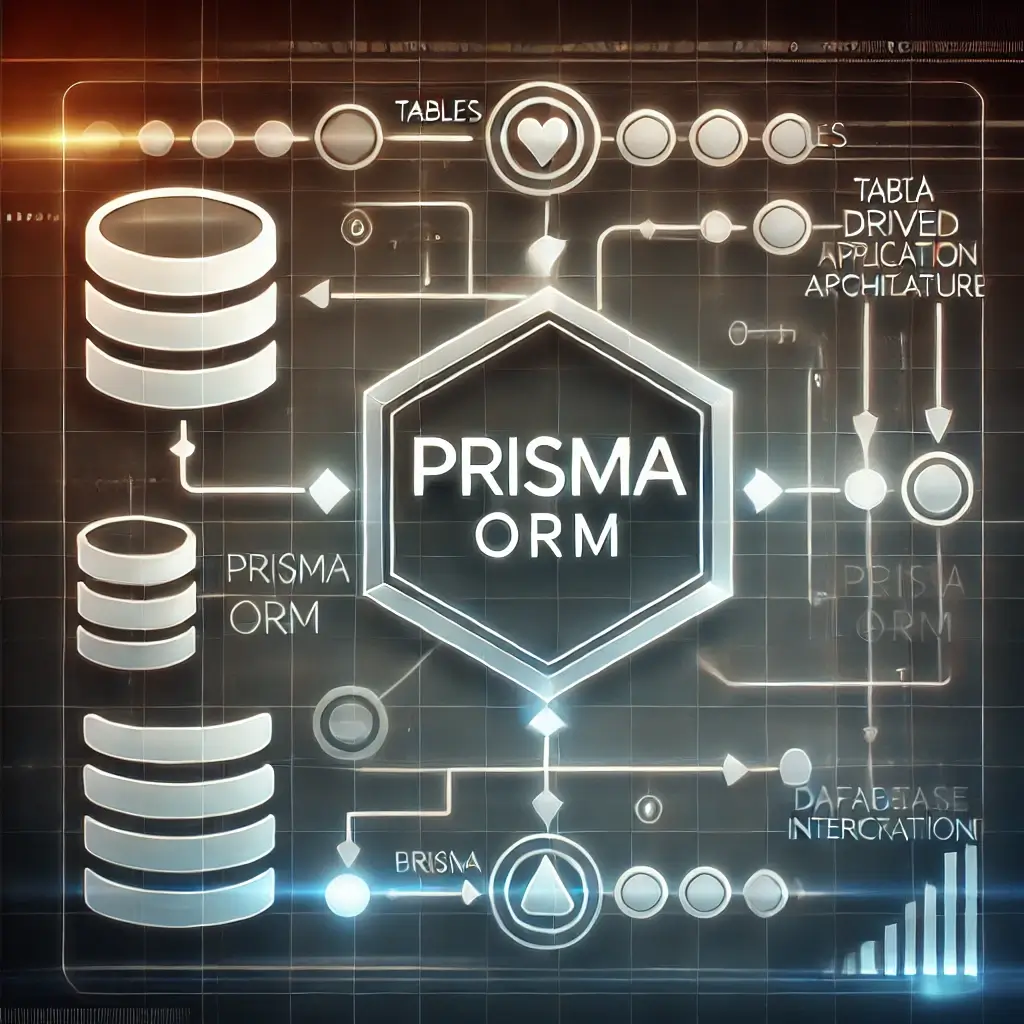
Mastering Prisma ORM with Real-World Scenarios: Building Scalable Applications with Ease
Choosing the right tools and frameworks in software development can significantly impact the performance, scalability, and maintainability of your applications. Prisma ORM, a next-generation Object-Relational Mapping tool for Node.js and TypeScript, has emerged as a powerful solution for modern database management. Unlike traditional ORMs, Prisma offers a fresh approach with its type-safe API, intuitive data modeling, and seamless integration with modern databases.
In this article, we’ll dive deep into Prisma ORM by exploring complex, real-world scenarios where Prisma’s unique features can be leveraged to solve challenging problems in web development. We’ll cover how Prisma can be used to build scalable, efficient, and maintainable applications in various contexts, from e-commerce to social media platforms.
1. Building a Scalable E-Commerce Platform
E-commerce platforms require a robust and scalable database structure to handle a large volume of transactions, product data, and user information. Let’s consider a scenario where you’re building a global e-commerce platform that needs to manage millions of products, thousands of concurrent users, and complex order processing logic.
Scenario Details:
- Product Catalog: Products can belong to multiple categories and have various attributes such as price, stock, size, and color.
- User Profiles: Users have profiles with purchase history, wish lists, and saved payment methods.
- Order Management: Orders can have multiple statuses (pending, shipped, delivered, canceled) and need to be linked with payment transactions and delivery tracking.
a. Type-Safe and Flexible Schema Modeling
Using Prisma, you can define complex relationships and data structures in a type-safe manner. Here’s an example of how you might define your data model:
model Product {
id Int @id @default(autoincrement())
name String
description String
price Float
stock Int
categories Category[] @relation(fields: [categoryId], references: [id])
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
model Category {
id Int @id @default(autoincrement())
name String @unique
products Product[]
}
model User {
id Int @id @default(autoincrement())
email String @unique
password String
orders Order[]
wishList Product[]
paymentInfo Json
}
model Order {
id Int @id @default(autoincrement())
status OrderStatus
user User @relation(fields: [userId], references: [id])
userId Int
products Product[]
createdAt DateTime @default(now())
}
enum OrderStatus {
PENDING
SHIPPED
DELIVERED
CANCELED
}
b. Efficient Data Retrieval with Nested Queries
Prisma allows you to perform complex nested queries to fetch data efficiently. For example, retrieving a user’s profile with all their past orders and wish list products in a single query:
const userProfile = await prisma.user.findUnique({
where: { id: userId },
include: {
orders: {
include: {
products: true,
},
},
wishList: true,
},
});
This approach minimizes database calls and improves the performance of your application, especially when dealing with a large dataset.
c. Handling Concurrency and Consistency
E-commerce platforms often face issues related to concurrency, such as multiple users trying to purchase the same item simultaneously. Prisma supports transactions, allowing you to handle such scenarios gracefully:
await prisma.$transaction(async (prisma) => {
const product = await prisma.product.findUnique({ where: { id: productId } });
if (product.stock < 1) {
throw new Error('Out of stock');
}
await prisma.product.update({
where: { id: productId },
data: { stock: product.stock - 1 },
});
await prisma.order.create({
data: {
userId,
products: { connect: { id: productId } },
status: 'PENDING',
},
});
});
2. Creating a Real-Time Social Media Platform
Developing a social media platform presents unique challenges, particularly around real-time data updates, complex relationships, and scalability. Consider building a platform that supports user-generated content, real-time notifications, and a recommendation engine.
Scenario Details:
- User Connections: Users can follow each other, forming a many-to-many relationship.
- Posts and Comments: Users can create posts and comment on others’ posts, necessitating a relational structure.
- Real-Time Features: Likes, comments, and new posts should trigger real-time updates.
a. Modeling Complex Relationships
Prisma allows you to model complex relationships like user connections and nested data for posts and comments:
model User {
id Int @id @default(autoincrement())
name String
email String @unique
posts Post[]
comments Comment[]
followers User[] @relation("UserFollowers", references: [id])
following User[] @relation("UserFollowing", references: [id])
}
model Post {
id Int @id @default(autoincrement())
content String
author User @relation(fields: [authorId], references: [id])
authorId Int
comments Comment[]
likes Like[]
createdAt DateTime @default(now())
}
model Comment {
id Int @id @default(autoincrement())
content String
post Post @relation(fields: [postId], references: [id])
postId Int
author User @relation(fields: [authorId], references: [id])
authorId Int
createdAt DateTime @default(now())
}
model Like {
id Int @id @default(autoincrement())
post Post @relation(fields: [postId], references: [id])
postId Int
user User @relation(fields: [userId], references: [id])
userId Int
createdAt DateTime @default(now())
}
b. Real-Time Data Handling with Prisma and GraphQL
Prisma works seamlessly with GraphQL, making it easier to implement real-time features. With subscriptions in GraphQL, you can push real-time updates to clients whenever a user likes a post or adds a comment:
type Subscription {
newLike(postId: Int!): Like
newComment(postId: Int!): Comment
}
You can use Prisma’s prisma.$subscribe
API with GraphQL to trigger these subscriptions and handle real-time updates efficiently.
c. Optimizing Read Performance with Efficient Queries
Social media platforms need to fetch large amounts of data efficiently, especially when loading a user’s feed. Prisma supports lazy loading and pagination, enabling you to fetch data as needed and manage performance:
const userFeed = await prisma.post.findMany({
where: { authorId: { in: followedUserIds } },
include: {
author: true,
comments: true,
likes: true,
},
take: 10,
skip: offset,
});
d. Scaling the Recommendation Engine with Prisma
A recommendation engine requires processing a significant amount of data to suggest friends or content. Prisma’s efficient query builder, combined with a service like Redis for caching, can help build a scalable recommendation system:
const recommendedUsers = await prisma.user.findMany({
where: {
AND: [
{ id: { notIn: currentUser.followingIds } },
{ id: { not: currentUser.id } },
],
},
orderBy: {
followers: 'desc',
},
take: 5,
});
3. Developing a SaaS Application with Multi-Tenancy
When building a Software-as-a-Service (SaaS) platform, multi-tenancy is a critical feature that allows multiple clients to use the same software instance while keeping their data isolated.
Scenario Details:
- Multi-Tenancy: Each client (tenant) should have isolated data, but all tenants share the same codebase.
- Role-Based Access Control (RBAC): Different users within a tenant have different roles (admin, editor, viewer) with varying levels of access.
a. Implementing Multi-Tenancy with Schema-Based Isolation
One approach to multi-tenancy is using separate schemas for each tenant. Prisma’s support for schema-based multi-tenancy makes it straightforward to configure different tenants within the same database:
model Tenant {
id Int @id @default(autoincrement())
name String
schema String @unique
users User[]
}
model User {
id Int @id @default(autoincrement())
name String
email String @unique
tenant Tenant @relation(fields: [tenantId], references: [id])
tenantId Int
role Role
}
enum Role {
ADMIN
EDITOR
VIEWER
}
Prisma allows you to connect to different schemas dynamically by changing the connection string or using database proxies.
b. Implementing Role-Based Access Control (RBAC)
With Prisma, enforcing RBAC is straightforward. You can define middleware to check user roles before executing queries:
prisma.$use(async (params, next) => {
if (params.model == 'User' && params.action == 'findMany') {
// Check if the user has the right role
if (!userHasRole('ADMIN')) {
throw new Error('Unauthorized');
}
}
return next(params);
});
c. Handling Data Isolation and Security
Prisma ensures data isolation by leveraging different schemas or row-level security. Additionally, Prisma integrates well with popular security libraries, allowing you to implement encryption and other security measures seamlessly.
Conclusion
Prisma ORM is not just another tool in your tech stack; it’s a powerful enabler for building complex, real-world applications with ease. By leveraging Prisma’s advanced features such as type-safe queries, efficient data retrieval, and flexible schema management, you can solve complex problems in various domains, from e-commerce and social media to SaaS platforms.
Whether you’re managing thousands of concurrent users or handling sensitive data across multiple tenants, Prisma’s modern approach to ORM can help you build scalable, maintainable, and high-performance applications. Dive into Prisma today, and start unlocking new possibilities for your next project!
Happy coding!