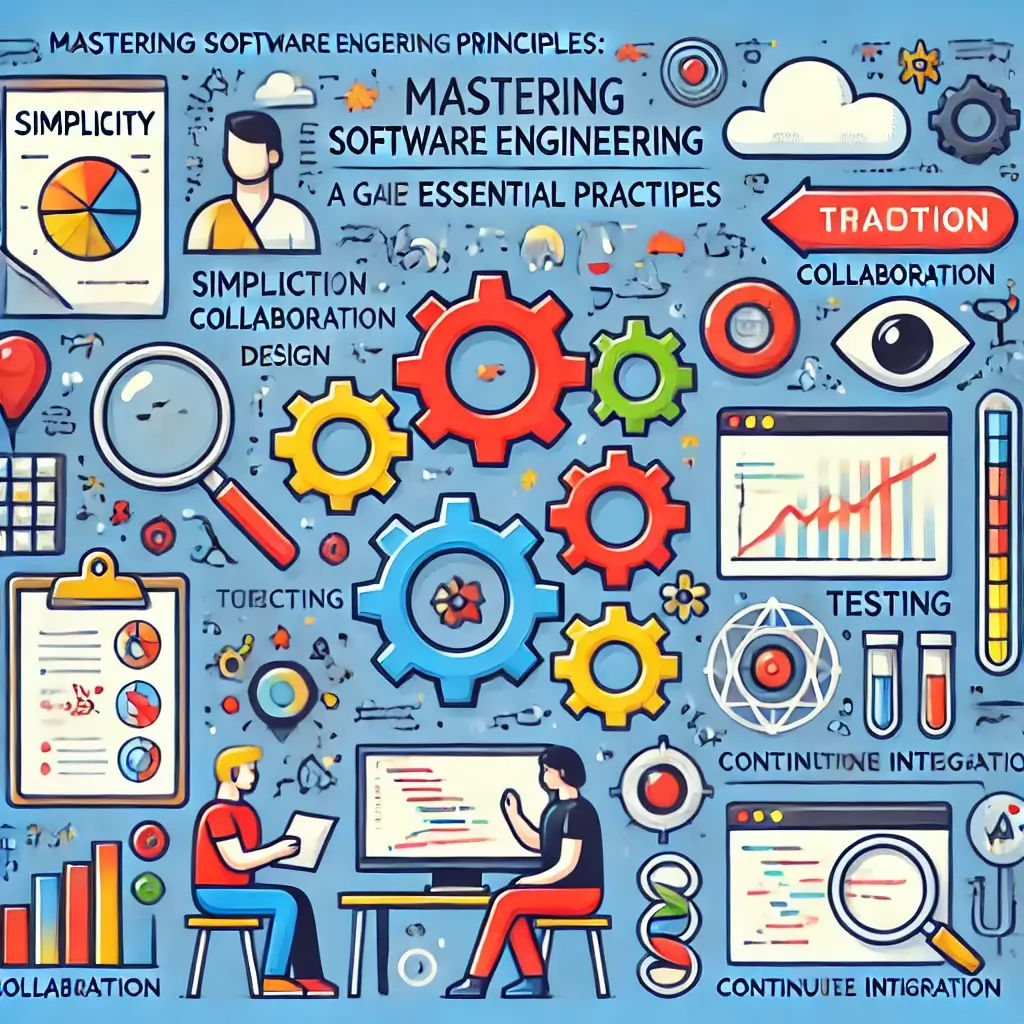
Learning Software Engineering Principles: A Guide to Essential Practices
In the dynamic world of software engineering, certain principles and practices stand out as foundational to building high-quality, maintainable, and scalable software. These principles, often referred to as best practices or key concepts, provide a roadmap for developers navigating the complexities of coding, architecture, and design. In this article, we will explore 15 essential software engineering principles and practices, such as KISS, YAGNI, DRY, SOLID, Agile, TDD, and more. Understanding and applying these concepts can help you become a more effective developer and create better software.
1. KISS: Keep It Simple, Stupid
KISS stands for Keep It Simple, Stupid. This principle stresses the importance of simplicity in software design and implementation. The core idea is to avoid unnecessary complexity in your code and solutions. Complex systems are harder to understand, maintain, and debug. By keeping things simple, you make your code more accessible to other developers and ensure that future changes are easier to manage.
Key Points of KISS:
- Write clear, straightforward code.
- Avoid over-engineering or anticipating future needs that may never materialize.
- Simple code is easier to test, maintain, and extend.
2. YAGNI: You Aren’t Gonna Need It
YAGNI stands for You Aren’t Gonna Need It. This principle encourages developers to avoid adding features or functionality until they are actually required. By focusing only on what is necessary for the present requirements, YAGNI helps reduce unnecessary complexity and prevents feature creep.
Key Points of YAGNI:
- Implement features only when they are needed.
- Helps keep the codebase lean and maintainable.
- Supports faster development by avoiding unnecessary work.
3. DRY: Don’t Repeat Yourself
DRY, or Don’t Repeat Yourself, is a principle aimed at reducing redundancy in code. It advocates for avoiding duplication by ensuring that each piece of knowledge or logic in the system has a single, authoritative representation.
Key Points of DRY:
- Write reusable code through functions, classes, or modules.
- Minimize the risk of errors by reducing the amount of code that needs to change when a concept is updated.
- Makes the codebase easier to maintain and refactor.
4. SOLID Principles
The SOLID principles are five design guidelines that help create more understandable, flexible, and maintainable object-oriented software:
- Single Responsibility Principle (SRP): A class should have only one reason to change, meaning it should have only one job or responsibility.
- Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types without altering the correctness of the program.
- Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules but on abstractions.
Key Points of SOLID:
- Promotes flexible and scalable software design.
- Reduces tight coupling and increases cohesion.
- Facilitates easier maintenance and enhances code readability.
5. Agile Methodology
Agile is a methodology that emphasizes iterative development, collaboration, and flexibility over rigid planning and processes. Agile focuses on delivering small, incremental improvements that add value to users, allowing teams to adapt quickly to changing requirements.
Key Points of Agile:
- Prioritizes customer collaboration and adaptability over following a fixed plan.
- Encourages iterative progress with regular feedback loops.
- Promotes teamwork, accountability, and continuous improvement.
6. TDD: Test-Driven Development
TDD stands for Test-Driven Development, a practice where developers write tests before writing the code that needs to pass those tests. TDD helps ensure that code meets its requirements and encourages writing smaller, more modular pieces of code.
Key Points of TDD:
- Encourages writing only the code necessary to pass the tests.
- Reduces bugs by catching them early in the development process.
- Promotes better design decisions and code structure through upfront planning.
7. BDD: Behavior-Driven Development
BDD stands for Behavior-Driven Development, an extension of TDD that focuses on the behavior of an application from the user’s perspective. BDD uses simple language to describe the desired behavior, making it accessible to non-developers.
Key Points of BDD:
- Aligns development with business needs by focusing on user-centric outcomes.
- Encourages writing acceptance tests before coding starts.
- Facilitates communication and collaboration between developers, testers, and business stakeholders.
8. DevOps
DevOps is a set of practices that combines software development (Dev) and IT operations (Ops). It aims to shorten the software development life cycle and deliver continuous integration and continuous deployment (CI/CD) with high quality.
Key Points of DevOps:
- Enhances collaboration between development and operations teams.
- Automates the deployment process for faster, more reliable releases.
- Improves efficiency and reduces errors by integrating practices like CI/CD.
9. CI/CD: Continuous Integration and Continuous Deployment
CI/CD stands for Continuous Integration and Continuous Deployment, practices that automate the testing and deployment of code. These practices ensure that code changes are consistently tested and deployed to production, reducing the risk of errors and accelerating delivery.
Key Points of CI/CD:
- Automates testing and deployment, reducing manual intervention.
- Facilitates frequent integration and feedback, leading to quicker identification of issues.
- Supports Agile methodologies by enabling rapid, reliable releases.
10. Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. The goal is to improve the code’s internal structure, making it easier to understand, maintain, and extend.
Key Points of Refactoring:
- Enhances code readability and reduces complexity.
- Identifies and eliminates code smells (indicators of potential problems).
- Supports adherence to principles like DRY, KISS, and SOLID.
11. MVP: Minimum Viable Product
MVP stands for Minimum Viable Product, a product development strategy focused on building the simplest version of a product that can still deliver value to users. This approach allows teams to quickly validate product ideas and gather user feedback.
Key Points of MVP:
- Validates product ideas with minimal investment.
- Helps prioritize features based on actual user needs and feedback.
- Encourages rapid iteration and learning.
12. Pair Programming
Pair Programming is an Agile technique where two developers work together at one workstation. One writes the code (the “driver”), while the other reviews it in real-time (the “observer” or “navigator”).
Key Points of Pair Programming:
- Encourages knowledge sharing and reduces the risk of errors.
- Improves code quality through continuous review.
- Enhances team collaboration and fosters mentorship.
13. Code Reviews
Code Reviews involve systematically examining code written by others to identify bugs, improve code quality, and ensure adherence to coding standards. It is a critical part of the development process that promotes collective ownership of the code.
Key Points of Code Reviews:
- Catch issues early in the development process before reaching production.
- Facilitate knowledge sharing and improve coding standards.
- Encourage collaborative problem-solving and continuous learning.
14. Modularity
Modularity is the design principle of breaking down a software system into separate, interchangeable components, each addressing a specific functionality. It supports easier maintenance, testing, and debugging.
Key Points of Modularity:
- Makes code easier to understand and maintain.
- Promotes reusability of components across different projects.
- Facilitates independent development and testing of modules.
15. Avoid Premature Optimization
Avoid Premature Optimization is a principle that cautions against optimizing code too early in the development process, especially before a clear understanding of where the bottlenecks are. Early optimization can lead to unnecessary complexity and divert focus from more critical issues.
Key Points of Avoiding Premature Optimization:
- Focus on writing clear and correct code before considering optimizations.
- Only optimize when performance bottlenecks are identified and are proven to impact the system significantly.
- Helps maintain code simplicity and readability until optimization is truly needed.
Conclusion
In software engineering, mastering these principles and practices is key to becoming a skilled developer and building robust, maintainable software. Principles like KISS, YAGNI, DRY, SOLID, Agile, TDD, BDD, DevOps, CI/CD, Refactoring, MVP, Pair Programming, Code Reviews, Modularity, and Avoiding Premature Optimization provide a comprehensive framework for effective software development. By incorporating these concepts into your workflow, you can ensure that your code is clean, efficient, and adaptable to future changes, ultimately leading to more successful projects and happier users.